A fundamental feature in C++ programming is the ability to loop. C++ offers three looping constructs in C++. The while loop has the following format:
Dec 19, 2008 Relaxing JAZZ For WORK and STUDY - Background Instrumental Concentration JAZZ for Work and Study - Duration: 2:13:09. Relax Music Recommended for you. To use a sequence of values e.g. A user may display a set of natural numbers from 1 to 10. There are three types of loops in C: While loop; For loop; Do-while loop. It is the simplest loop of C. This loop executes one or more statements while the given condition remains true. It is useful where the number of iteration are not. When condition returns false, the control comes out of loop and jumps to the next statement in the program after while loop. Note: The important point to note when using while loop is that we need to use increment or decrement statement inside while loop so that the loop variable gets changed on each iteration, and at some point condition returns false.
How To Use While Loop In Dev C In Windows 10
When a program comes upon a while loop, it first evaluates the expression in the parentheses. If this expression is true, then control passes to the first line inside the {. When control reaches the }, the program returns back to the expression and starts over. Control continues to cycle through the codein the braces until expression evaluates to false (or until something else breaks the loop).
The following Factorial program demonstrates the while loop:
How To Use While Loop In Dev C In Excel
The program starts by prompting the user for a target value. The program reads this value into nTarget. The program then initializes both nAccumulator and nValue to 1 before entering the loop.
(Pay attention — this is the interesting part.) The program compares nValue to nTarget. Assume that the user had entered a target value of 5. On the first loop, the question becomes, “Is 1 less than or equal to 5?” The answer is obviously true, so control flows into the loop.
The program outputs the value of nAccumulator (1) and nValue (also 1) before multiplying nAccumulator by nValue and storing the result back into nAccumulator.
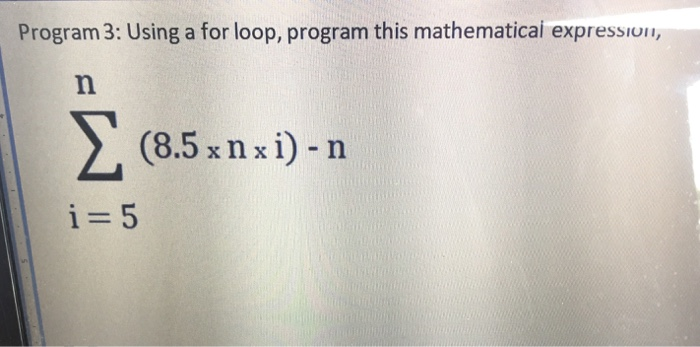
The last statement in the loop increments nValue from 1 to 2.
That done, control passes back up to the while statement where nValue (now 2) is compared to nTarget (still 5). “Is 2 less than or equal to 5?” Clearly, true; so control flows back into the loop. nAccumulator is now set to the result of nAccumulator (1) times nValue (2). The last statement increments nValue to 3.

This cycle of fun continues until nValue reaches the value 6, which is no longer less than or equal to 5. At that point, control passes to the first statement beyond the closed brace }. This is shown graphically here.
If While Loop In C
The actual output from the program appears as follows for an input value of 5:

You are not guaranteed that the code within the braces of a while loop is executed at all: If the conditional expression is false the first time it’s evaluated, control passes around the braces without ever diving in. Consider, for example, the output from the Factorial program when the user enters a target value of 0:
No lines of output are generated from within the loop because the condition “Is nValue less than or equal to 0” was false even for the initial value of 1. The body of the while loop was never executed.